Custom Fields
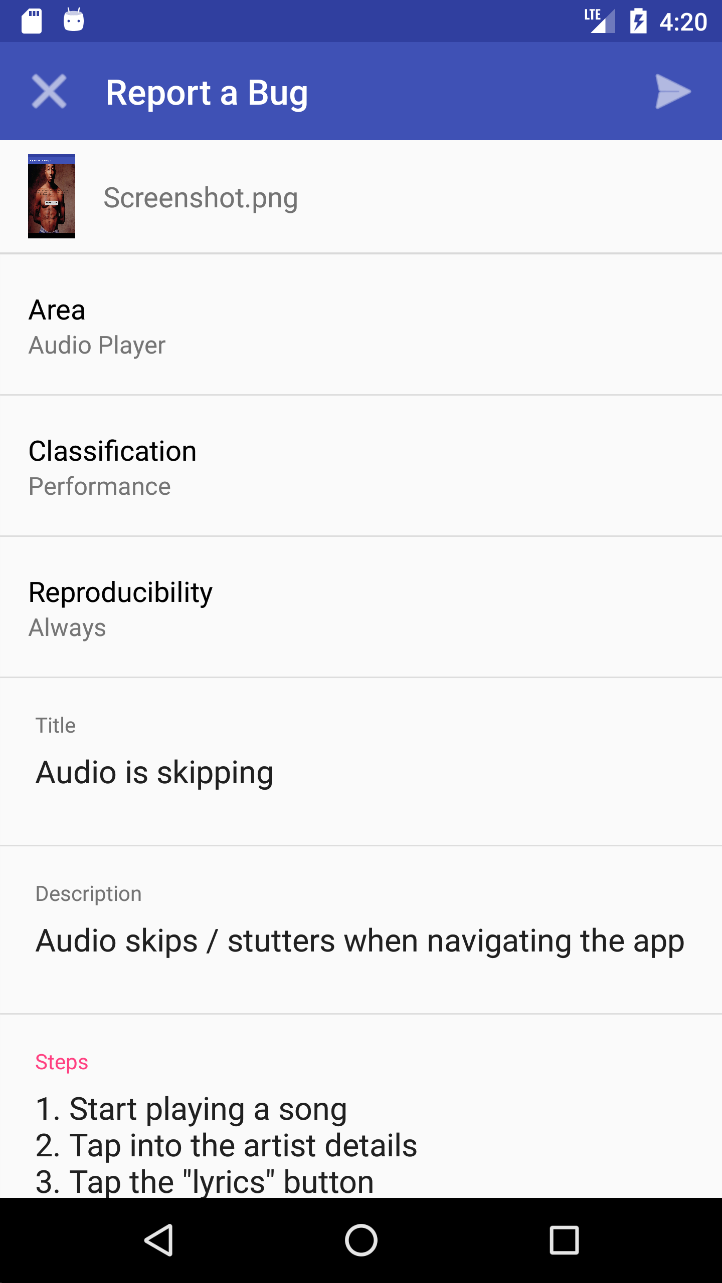
The Buglife bug reporter UI can be configured to present custom input fields. There are two types of available input fields to choose from: Text input fields and Picker input fields.
To configure custom input fields, initialize one or more instances of TextInputField
and/or PickerInputField
, and configure Buglife with them using Buglife.setInputFields()
.
Text input fields
The TextInputField
class can be used to configure text entry fields, and can be single- or multi-line. You may choose to configure custom text input fields to collect information such as steps to reproduce, or information about a user.
TextInputField stepsField = new TextInputField("Steps");
stepsField.setMultiline(true);
Buglife.setInputFields(stepsField);
The "Summary" input field
By default, the Buglife bug reporter is configured with the "Summary" input field (typically labeled with "What happened?"), which is a special instance of type TextInputField
.
To use the special Summary field with custom fields, grab an instance of it using TextInputField.summaryInputField()
.
// The following is equivalent to simply using the default Buglife configuration
TextInputField summaryField = TextInputField.summaryInputField();
Buglife.setInputFields(summaryField);
Picker input fields
The PickerInputField
class can be used to surface "pickers", which allow users to select a single value from an array of options.
PickerInputField reproducibilityField = new PickerInputField("Area");
reproducibilityField.addOption("Music library");
reproducibilityField.addOption("Audio player");
reproducibilityField.addOption("Downloads");
reproducibilityField.addOption("Search");
Buglife.setInputFields(reproducibilityField);
Default values
You can configure default values for both text & picker input fields using Buglife.putAttribute()
. For example, you can use this to dynamically configure the default value for a field when the user switches screens in your app.
The attribute name should equal one of your input field names; If setting an attribute for a picker, the attribute value should equal one of that picker's options.
// For the above picker example,
// this will select the "Audio player" option by default
Buglife.putAttribute("Area", "Audio player");
Example
The following example configures the bug reporter UI with 3 input fields: the "Summary" field, a "Reproducibility" picker, and a multi-line "Steps" field:
TextInputField summaryField = TextInputField.summaryInputField();
PickerInputField reproducibilityField = new PickerInputField("Reproducibility");
reproducibilityField.addOption("Always");
reproducibilityField.addOption("Sometimes");
reproducibilityField.addOption("Rarely");
TextInputField stepsField = new TextInputField("Steps");
stepsField.setMultiline(true);
Buglife.setInputFields(summaryField, reproducibilityField, stepsField);